Commenting your code
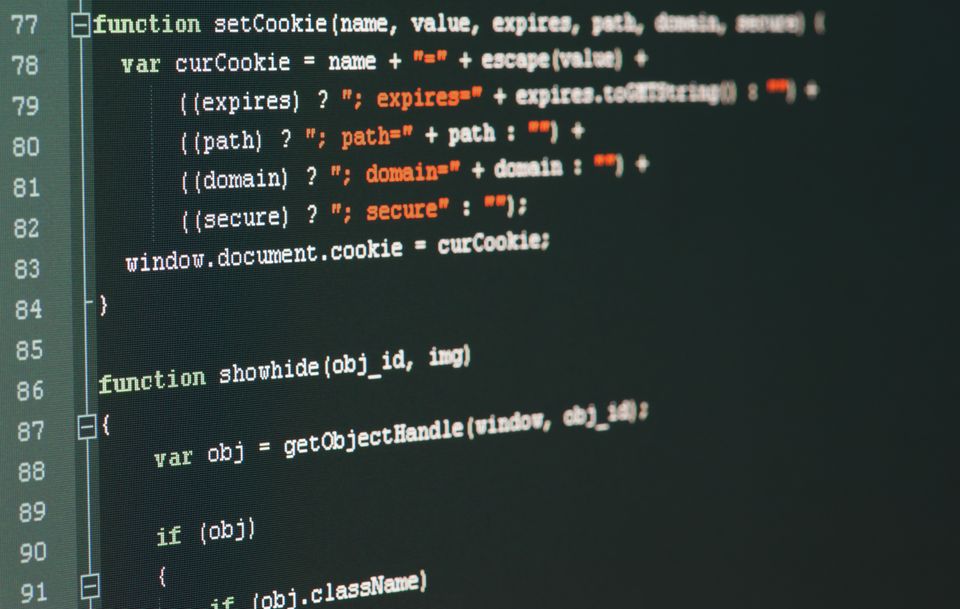
It is generally agreed upon that the code you write should be expressive enough that it is self documenting. It means that your code should be clear enough that you don't need to write comments on it.
In practice however, I've found that many developers I've worked with request comments that go along with the code. Below is one method from a few years ago that I was chatting with a team member about (note: this is just an example of the complexity of the code that we were discussing, this wasn't real application code).
# Orders a list of arrays, flatten the joined array, sorts, and then returns the result
def order_list
a = [3, 1, 5]
b = [4, 6, 2]
c = [[3, 5, 7], [1, 2, 4]]
# add arrays, flatten it, and sort it
(a + b + c).flatten.sort
end
The original code didn't have comments on it, but was added later on by another team member.
I think most developers will agree that the above method doe snot need to be commented on at all. The name of the method clearly states what it is intending to do, and ruby methods are generally named descriptive enough to to signify to the developer what the flatten
and the sort
method should do. There is absolutely no reason to comment this method.
The computer doesn't care about your comments
The comments you write are for other humans, and... it's written by humans. Which means that we'll forget to update our comments as we update our code.
Software projects have to constantly chance to meet changing business needs. If your code depends on your comments to make sense, and the functionality of the code changes over time, someone will inevitably forget to update the comment that describes wheat the code is doing. And yes, your code will run even if you forget to update the comment, but the next developer who comes into the project will inevitably end up reading the outdated comments and misunderstand what the code is doing. You can see how this can cause all sorts of confusion as the software and the team grows.
You can prevent this by writing self documenting code.
When to comment code
There are times when comments can actually help. The main situation is when the code is orchestrating some business rules with exceptions that may not be obvious to the developer.
For example, let's say that there's a method in your app that calculates the average of two numbers. Normally, you would sum the two numbers and divide the result by 2. But let's say for example, you find that the method you're looking at is dividing the result by 5. If there aren't any comments, you would think that this is a bug. But what if it isn't a bug and is intentionally written this way due to some business rule that's specific to the industry you're working in. This would be a great time to comment your code.
def average_of_numbers(numbers)
# Divides by four more than the size of the list due to business rule
numbers.inject(:+) / (numbers.size + 4)
end
If the comment wasn't there, the method looks incorrectly written. Without the comment, you might try to fix it, unintentionally breaking the app. In this case, commenting is beneficial.
Try to write self descriptive code. Only comment when it truly adds value.